Introduction
Strong Foundation in Java for Job Support
In present day aggressive process market, having a sturdy basis in Java programming is essential for securing profitable possibilities inside the tech enterprise. Java, being one of the most extensively used programming languages, powers numerous applications, ranging from agency software program to cell apps and web development. Employers regularly searching for applicants with proficiency in Java because of its versatility, reliability, and scalability.
Overview of What Constitutes a Solid Foundation in Java
Building a stable basis in Java entails mastering fundamental ideas and principles that form the spine of the language. This includes knowledge object-oriented programming (OOP) standards along with inheritance, polymorphism, encapsulation, and abstraction. Additionally, talent in dealing with statistics systems, inclusive of arrays, lists, stacks, and queues, is vital for efficient algorithm implementation and trouble-fixing. Furthermore, familiarity with middle Java APIs, exception managing, multithreading, and input/output operations is essential for developing strong and green Java packages.
Objectives of the Blog
The primary goals of this blog are:
- To offer readers with a comprehensive know-how of the fundamental ideas of Java programming.
- To equip readers with important abilities and expertise required to build sturdy Java packages.
- To offer practical hints, tutorials, and resources for reinforcing talent in Java programming.
- To highlight the importance of a strong basis in Java for profession advancement and job assist.
- To address not unusual challenges and misconceptions faced by using rookies even as learning Java programming.
- To encourage and inspire readers to embark on a adventure toward turning into proficient Java builders.
Throughout this blog, we will delve deep into numerous aspects of Java programming, offering insights, examples, and sensible sporting activities to useful resource readers in strengthening their Java capabilities and advancing their careers in the ever-evolving tech enterprise. Stay tuned for insightful discussions and tasty content material to be able to empower you on your Java programming journey.
Understanding the Basics
Introduction to Java Programming Language
Java, advanced by way of Sun Microsystems (now owned by using Oracle Corporation), is a high-degree, item-oriented programming language famed for its platform independence and flexibility. It became released in 1995 and has considering that emerge as one of the maximum popular programming languages globally, particularly for employer-level programs and internet development. Java’s slogan “Write Once, Run Anywhere” displays its capacity to run on any tool with the Java Virtual Machine (JVM), making it fantastically transportable.
Key Features and Benefits of Java
- Platform Independence: Java packages are compiled into bytecode, which may be performed on any platform with a JVM, irrespective of the underlying hardware and running system.
- Object-Oriented: Java follows the standards of item-oriented programming (OOP), emphasizing modularity, reusability, and encapsulation.
- Robust and Secure: Java’s strong memory control, exception dealing with, and integrated safety features make it strong and stable for growing challenge-critical packages.
- Multi-threading Support: Java gives integrated support for multithreading, permitting concurrent execution of a couple of duties inside a unmarried program.
- Rich Standard Library: Java comes with an intensive fashionable library (Java API) that provides geared up-to-use instructions and techniques for numerous tasks, simplifying development.
- Automatic Garbage Collection: Java’s automatic garbage series mechanism manages reminiscence allocation and deallocation, relieving developers from guide reminiscence management tasks.
- Dynamic and Extensible: Java supports dynamic loading of classes and runtime reflection, enabling dynamic and extensible applications.
- Community Support: Java boasts a good sized and energetic developer network, offering ample sources, libraries, and frameworks for speedy improvement.
Basic Syntax and Structure
Java programs are prepared into instructions, which contain strategies and fields. Each Java application have to have at least one elegance with a main() technique as the entry point. Java syntax is much like C and C++, making it easy for developers familiar with these languages to transition to Java. Statements in Java are terminated by means of a semicolon (;), and code blocks are enclosed inside curly braces ().
Data Types and Variables
Java helps numerous facts kinds, including primitive sorts (e.g., int, double, boolean) and reference kinds (e.g., items, arrays). Variables are used to shop information, and their kinds must be declared before use. Java employs sturdy typing, that means variables are strictly typed and have to be compatible with the assigned statistics type.
Control Flow Statements (if-else, Loops)
Java presents manage go with the flow statements such as if-else for conditional branching and loops like for, whilst, and do-while for iterative execution. These statements permit builders to govern the float of application execution based on situations or to copy a block of code a couple of instances.
Understanding those fundamental concepts lays the groundwork for studying Java programming and building sophisticated applications. In the subsequent sections, we can discover each of those subjects in detail, observed via examples and realistic sporting activities to reinforce studying.
Object-Oriented Programming (OOP) Concepts
Explanation of OOP Principles
Object-Oriented Programming (OOP) is a programming paradigm based on the idea of “items,” that can incorporate statistics in the form of fields (attributes or houses) and code in the form of techniques (techniques or features). OOP revolves round 4 primary standards:
Encapsulation: Encapsulation refers to the bundling of statistics and methods that function on that statistics right into a unmarried unit, referred to as a class. This precept enables in hiding the internal state and conduct of an item from the outdoor international and most effective exposing the vital interfaces for interaction.
Inheritance: Inheritance is a mechanism by way of which a new elegance (subclass or derived class) is made out of an existing elegance (superclass or base elegance). The subclass inherits attributes and strategies from its superclass and also can have its own additional attributes and techniques. This helps code reusability and promotes the idea of hierarchical classification.
Polymorphism: Polymorphism lets in items of different classes to be treated as objects of a commonplace superclass. It allows a unmarried interface for use for entities of different sorts and offers flexibility in method invocation. Polymorphism is accomplished via method overriding and method overloading.
Abstraction: Abstraction entails simplifying complex systems through representing the crucial features whilst hiding the implementation details. In OOP, abstraction is carried out through abstract classes and interfaces, which define a blueprint for training without specifying their specific implementation.
Classes and Objects
In OOP, a category is a blueprint or template for developing items. It defines the shape and conduct of gadgets with the aid of specifying attributes (fields) and strategies. Objects are instances of lessons, representing real-global entities with a specific country and behavior. Multiple items may be produced from the equal magnificence, each having its personal set of attributes and strategies.
Inheritance and Polymorphism
Inheritance permits lessons to inherit attributes and techniques from other classes, promoting code reuse and organising hierarchical relationships. Subclasses inherit traits from their superclass and can override inherited methods to provide specialised behavior. Polymorphism permits items to showcase different behaviors primarily based on their statistics sorts or classes, fostering flexibility and extensibility in code.
Encapsulation and Abstraction
Encapsulation bundles statistics and techniques within a class, controlling access to the inner nation of objects and shielding them from external interference. It enhances modularity, maintainability, and safety by imposing facts hiding and statistics hiding. Abstraction makes a speciality of defining important functions whilst hiding implementation info, allowing builders to paintings at better tiers of abstraction without being involved with low-stage implementation specifics.
Importance of OOP in Java Development
OOP is fundamental to Java improvement because the language itself is designed round OOP ideas. Java’s big use of instructions, gadgets, inheritance, polymorphism, encapsulation, and abstraction facilitates modular and maintainable code. OOP promotes code reusability, scalability, and versatility, making it simpler to design and control complex systems. Understanding OOP concepts is essential for leveraging Java’s complete potential and growing sturdy, scalable, and maintainable applications.
Core Java Libraries
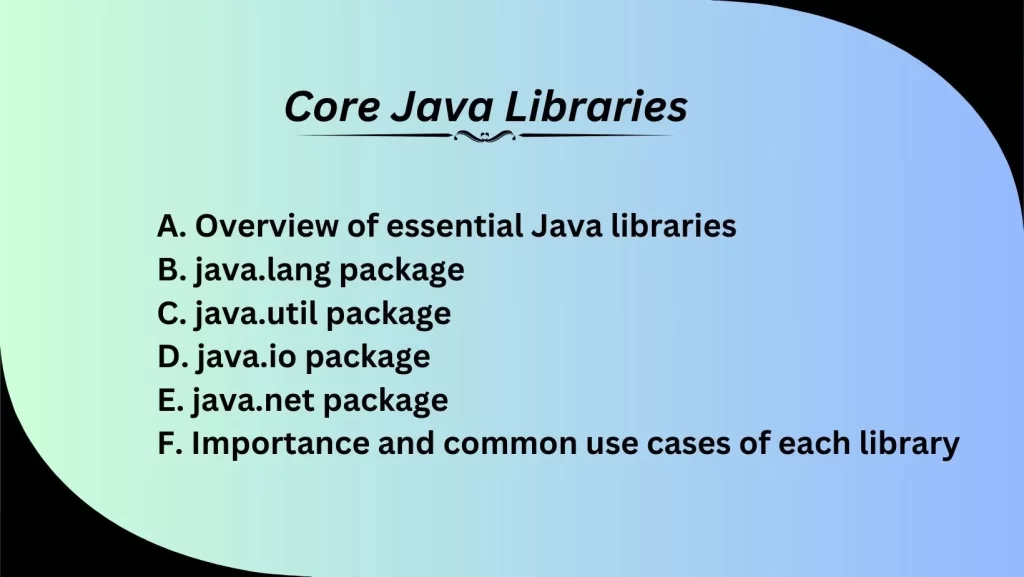
Overview of Essential Java Libraries
Java offers a wealthy set of general libraries that cover a extensive range of functionalities, making development faster and more efficient. Some crucial Java libraries consist of:
java.Lang: Provides fundamental lessons and functionalities which includes simple records types, math operations, string manipulation, and system operations.
Java.Util: Contains software instructions and information structures for managing collections, date and time, random quantity era, and other generally used functionalities.
Java.Io: Offers training for enter and output operations, including report handling, circulate coping with, serialization, and compression.
Java.Internet: Facilitates network programming through presenting classes for networking protocols, socket programming, URL dealing with, and communique over the internet.
Java.Lang Package
The java.Lang package deal is routinely imported into each Java software and carries fundamental lessons and functionalities important for basic Java programming. It consists of lessons for primary information kinds (e.G., Integer, Double), math operations, string manipulation (String), gadget operations (System), and exception coping with (Throwable).
Java.Util Package
The java.Util bundle provides software classes and information structures to handle collections, date and time, random variety technology, and other application functionalities. Commonly used classes on this bundle consist of ArrayList, HashMap, Date, Calendar, Random, and Scanner. These lessons are extensively utilized in Java programming for facts manipulation and management.
Java.Io Package
The java.Io package deal includes classes for input and output operations, together with record handling, move handling, serialization, and compression. Key instructions in this bundle include FileInputStream, FileOutputStream, FileReader, FileWriter, ObjectInputStream, ObjectOutputStream, and various movement classes (e.g., InputStream, OutputStream).
Java.Net Package
The java.Net package facilitates community programming in Java through imparting training for networking protocols, socket programming, URL managing, and communique over the net. Notable classes on this package consist of URL, HttpURLConnection, Socket, ServerSocket, DatagramSocket, and InetAddress. These instructions enable builders to create network-aware applications for conversation between computers over the internet or local networks.
Importance and Common Use Cases of Each Library
java.Lang: Essential for primary Java programming, which includes running with primary statistics kinds, strings, device operations, and exception dealing with.
Java.Util: Widely used for facts manipulation and control, which includes coping with collections, dates and instances, random wide variety generation, and input/output operations.
Java.Io: Crucial for report and move dealing with, serialization, and compression, regularly utilized in report processing, data patience, and community communication.
Java.Net: Essential for network programming, permitting verbal exchange among computer systems over the net or nearby networks, facilitating internet development, customer-server packages, and community-primarily based services.
Understanding and efficiently making use of those center Java libraries is vital for developing robust and efficient Java applications across numerous domain names.
Exception Handling
Understanding Exceptions in Java
In Java, an exception is an occasion that disrupts the normal go with the flow of a software’s execution. It usually happens while a mistakes situation arises at some stage in runtime, along with department by means of 0, invalid enter, or report no longer located.
Types of Exceptions
Exceptions in Java are categorised into sorts: checked exceptions and unchecked exceptions. Checked exceptions are checked at bring together time and must be either handled the usage of attempt-catch blocks or declared inside the technique signature the usage of the throws key-word. Unchecked exceptions, then again, are not checked at assemble time and consist of runtime exceptions and errors.
Strive-catch Blocks
Try-trap blocks are used to deal with exceptions in Java. The strive block consists of the code which could throw an exception, at the same time as the capture block catches and handles the exception if it occurs. Multiple catch blocks may be used to handle one-of-a-kind sorts of exceptions, and a finally block may be used to execute cleanup code irrespective of whether or not an exception takes place or now not.
Exception Propagation
When an exception takes place in a way, it could be propagated up the decision stack till it is stuck and dealt with via an appropriate catch block. If an exception is not stuck inside a technique, it’s far propagated to the calling technique, and the procedure maintains until a seize block is located or the exception reaches the top-level caller, ensuing in software termination.
Best Practices for Exception Handling
Handle exceptions at the best level of abstraction, near wherein they arise, to maintain code readability and maintainability.
Use unique exception sorts to provide significant error messages and improve blunders analysis and recovery.
Favor checked exceptions for great conditions that may be reasonably predicted and recovered from, and use unchecked exceptions for programming mistakes or situations that cannot be recovered from.
Always release sources (e.g., document handles, database connections) in a finally block to ensure proper cleanup, even though an exception happens.
Use try-with-sources statements to mechanically close resources in a safe and efficient way, added in Java 7.
By following those first-rate practices, developers can write sturdy and maintainable code with powerful exception coping with, improving the reliability and balance of Java packages.
Collections Framework
Introduction to Collections in Java
The Collections Framework in Java affords a fixed of interfaces, implementations, and algorithms to govern and store collections of gadgets correctly. Collections are used to institution a couple of elements right into a single unit and provide diverse operations for getting access to, manipulating, and iterating over these elements.
Lists, Sets, and Maps
Lists: Lists maintain an ordered collection of factors, allowing reproduction factors and imparting indexed get entry to elements. Common implementations include ArrayList and LinkedList.
Sets: Sets constitute a group of unique elements with no duplicates. Implementations include HashSet, TreeSet, and LinkedHashSet.
Maps: Maps keep key-value pairs and provide green retrieval of values based totally on keys. Implementations encompass HashMap, TreeMap, LinkedHashMap, and ConcurrentHashMap.
Iteration and Manipulation of Collections
Java presents various strategies for iterating over and manipulating collections, consisting of iterators, more suitable for loops (for-every loops), and circulate APIs brought in Java eight. These methods permit developers to carry out common operations like including, getting rid of, looking, and sorting factors in collections.
Sorting and Searching
The Collections Framework consists of application methods for sorting and looking factors in collections. Sorting may be finished the usage of the Collections.Sort() technique, which uses natural ordering or a custom comparator. For searching, techniques like Collections.BinarySearch() can be used on looked after collections to correctly discover factors.
Performance Considerations
When working with collections, it’s crucial to keep in mind performance implications, specifically for huge datasets. Choosing the right series implementation primarily based on the necessities (e.G., get entry to styles, concurrency) can considerably effect overall performance. Additionally, knowledge time complexities of operations (e.g., insertion, deletion, looking) allows in choosing the most efficient information shape for a given state of affairs.
Multithreading and Concurrency
Basics of Multithreading
Multithreading includes executing multiple threads simultaneously within a unmarried process, permitting programs to carry out a couple of responsibilities simultaneously. Threads are lightweight methods that percentage the equal memory space however have their own execution glide.
Creating and Managing Threads
In Java, threads can be created through extending the Thread magnificence or implementing the Runnable interface. Threads are started out using the start() approach and may be managed the use of techniques like sleep(), be part of(), and interrupt().
Synchronization and Locks
Concurrency problems inclusive of race situations and records inconsistency can rise up whilst multiple threads get entry to shared resources concurrently. Synchronization mechanisms like synchronized blocks and locks (e.g., ReentrantLock) are used to coordinate get entry to shared resources and prevent concurrent get right of entry to violations.
Concurrent Data Structures
Java offers thread-secure implementations of common records systems within the java.Util.Concurrent package deal, inclusive of ConcurrentHashMap, ConcurrentLinkedQueue, and CopyOnWriteArrayList. These facts structures are designed to assist concurrent get entry to with out express synchronization and are optimized for overall performance in multithreaded environments.
Best Practices for Writing Concurrent Code
Minimize shared mutable kingdom: Reduce the use of shared mutable variables to keep away from race conditions and statistics inconsistency.
Use thread-secure records systems: Prefer thread-secure collections and concurrent information systems to address shared resources in multithreaded environments.
Avoid needless synchronization: Use fine-grained locking and synchronization best where important to limit contention and improve concurrency.
Design for concurrency: Design software program with concurrency in mind, thinking about thread protection, synchronization, and performance implications from the outset.
File Handling and I/O Operations
Reading from and Writing to Files
Java presents instructions like File, FileInputStream, FileOutputStream, FileReader, and FileWriter for reading from and writing to documents. These instructions help diverse record operations together with analyzing/writing bytes or characters, creating, deleting, and traversing directories.
Working with Streams
Streams are used to examine from or write to files efficiently in Java. Buffered streams (BufferedReader, BufferedWriter) can improve I/O overall performance through decreasing the quantity of device calls.
File Handling Best Practices
Close resources nicely: Always close file streams and other I/O assets the usage of strive-with-sources or in a finally block to launch system resources.
Handle exceptions gracefully: Use exception handling to deal with file I/O mistakes effectively and offer suitable blunders messages or fallback mechanisms.
Use green I/O operations: Prefer buffered I/O streams for better overall performance, particularly while dealing with big documents or frequent I/O operations.
Exception Handling in File Operations
File operations in Java can throw diverse exceptions, consisting of FileNotFoundException, IOException, and SecurityException. Proper exception handling is critical to address those mistakes gracefully, near assets competently, and provide significant comments to customers in case of mistakes.
JDBC (Java Database Connectivity)
Introduction to JDBC
JDBC (Java Database Connectivity) is a Java API that enables Java packages to have interaction with databases. It offers a standard interface for gaining access to and manipulating relational databases, permitting developers to execute SQL queries, retrieve consequences, and control database transactions from Java code.
Connecting to Databases
To connect to a database using JDBC, builders want to load and register the appropriate database driving force, establish a connection the use of connection parameters such as URL, username, and password, and obtain a connection object the usage of DriverManager.GetConnection() approach.
Executing SQL Queries
JDBC allows developers to execute SQL queries towards the linked database using Statement, PreparedStatement, and CallableStatement items. These objects can be used to execute SQL statements, retrieve end result units, and carry out database updates.
Handling Database Transactions
Database transactions ensure data consistency and integrity by way of grouping multiple database operations right into a single unit of labor that either succeeds or fails as an entire. JDBC gives support for handling transactions the use of methods like setAutoCommit(), dedicate(), and rollback() on connection objects.
Best Practices for Using JDBC
Use connection pooling: Implement connection pooling to improve performance and scalability via reusing database connections.
Use PreparedStatement: Prefer PreparedStatement over Statement for executing parameterized SQL queries to prevent SQL injection assaults and improve overall performance.
Close sources well: Always close JDBC sources (e.G., connections, statements, end result sets) in ultimately blocks or using attempt-with-sources to launch database sources and prevent memory leaks.
Handle exceptions gracefully: Use exception managing to deal with database-associated mistakes and offer suitable errors messages or fallback mechanisms to users.
Follow transaction control exceptional practices: Use transactions correctly to make certain information consistency and integrity, handle exceptions inside transactions, and roll returned transactions on failure.
Testing and Debugging
Importance of Testing in Java Development
Testing is essential in Java development to ensure the reliability, capability, and performance of software packages. It facilitates pick out insects, validate software program necessities, and enhance code nice via detecting defects early in the improvement lifecycle.
Unit Testing with JUnit
JUnit is a famous Java testing framework used for writing and executing unit checks. It affords annotations, assertions, and test runners to outline and run check cases, verify expected behavior, and automate the checking out method.
Debugging Techniques and Tools
Java builders use numerous debugging techniques and equipment to identify and remedy software defects efficaciously. Debugging tools like IDE debuggers (e.g., IntelliJ IDEA, Eclipse), logging frameworks (e.g., Log4j, SLF4J), and tracking equipment (e.g., JConsole, VisualVM) assist developers diagnose and connect problems throughout development and trying out.
Code Review and Quality Assurance Practices
Code assessment and quality assurance practices contain systematic exam of code by means of peers to identify defects, make certain adherence to coding standards, and improve universal code exceptional. Code evaluate equipment (e.G., Gerrit, Crucible) and static evaluation equipment (e.G., SonarQube, FindBugs) assist automate and streamline code evaluate and satisfactory assurance techniques.
Looking for Java job support from India? Our comprehensive services ensure you master Java fundamentals, including core libraries, object-oriented programming, and JDBC, setting you up for success in the competitive job market. With our guidance, you’ll gain the skills and confidence needed to excel in Java development roles.
Conclusion
Recap of Key Points Covered
Throughout this manual, we included essential topics in Java programming, consisting of core Java libraries, item-orientated programming standards, multithreading, JDBC, checking out, and debugging. We discussed fundamental standards, best practices, and practical techniques for growing strong, green, and maintainable Java packages.
Importance of Mastering Java Fundamentals for Job Support
Mastering Java fundamentals is crucial for securing activity opportunities, advancing career possibilities, and turning into proficient in Java development. A strong basis in Java programming enables builders to tackle complicated demanding situations, construct scalable solutions, and stay competitive in the ever-evolving tech industry.
Encouragement for Further Exploration and Practice
As you preserve your journey in Java programming, I encourage you to explore advanced topics, test with new technology, and have interaction in non-stop getting to know and exercise. Stay curious, keep honing your abilities, and leverage the considerable resources to be had in the Java network to develop as a gifted and versatile Java developer.