Introduction
Explanation of Authentication and Authorization
Authentication refers to the process of verifying the identity of a user or system attempting to access a resource. It ensures that the user is who they claim to be. Authorization, on the other hand, determines what actions an authenticated user is allowed to perform within a system or on a resource. Together, these processes form the foundation of security protocols in software applications, ensuring that only authorized users can access specific features or data.
Importance of Handling Authentication and Authorization in Selenium
In the context of Selenium, which is primarily used for automated testing of web applications, handling authentication and authorization is crucial for accurately simulating user interactions. Many web applications require users to log in with valid credentials before accessing certain functionalities or data. Failing to address authentication and authorization mechanisms in Selenium scripts can lead to incomplete or inaccurate test results. Therefore, understanding how to authenticate users and manage authorization levels within Selenium tests is essential for ensuring the reliability and effectiveness of the testing process.
Purpose and Scope of the Guide
The purpose of this guide is to provide comprehensive instructions and best practices for handling authentication and authorization in Selenium test automation. It will cover various scenarios, such as basic authentication, form-based authentication, OAuth, and role-based access control (RBAC). Additionally, the guide will address common challenges and pitfalls encountered when dealing with authentication and authorization in Selenium scripts, along with strategies for overcoming them. By following the guidelines outlined in this guide, testers and developers can enhance the robustness and accuracy of their Selenium test suites while effectively simulating real-world user interactions in authenticated environments.
Understanding Authentication
Definition and Concept
Authentication is the process of verifying the identity of a user or system, ensuring that they are who they claim to be. It involves presenting credentials, such as usernames and passwords, to a system, which then validates these credentials against stored records. Successful authentication grants the user access to the system or specific resources within it, while failed authentication results in denial of access.
Types of Authentication Mechanisms
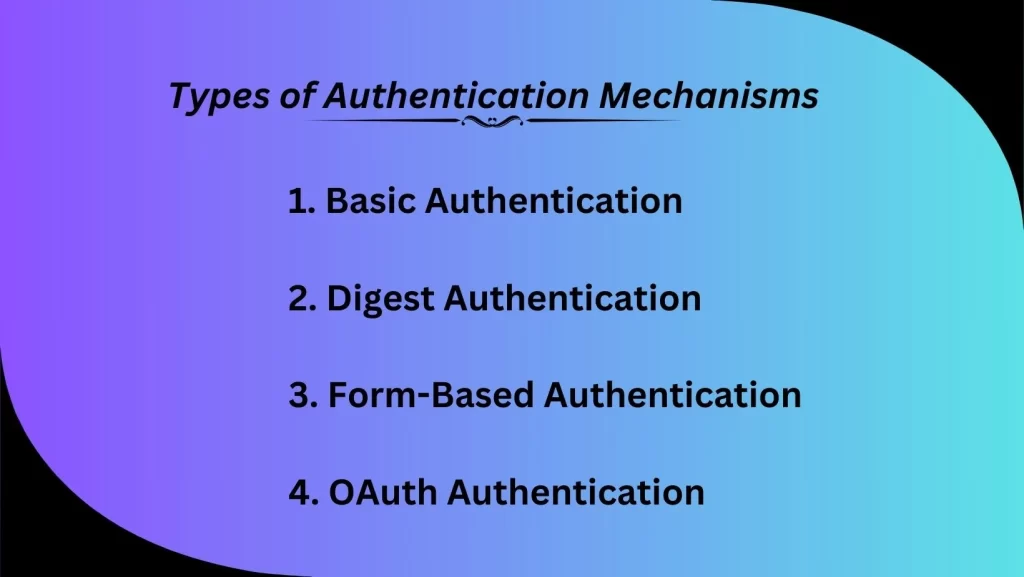
Basic Authentication
Basic authentication is a simple mechanism where the user’s credentials (typically a username and password) are sent as plaintext in the HTTP request headers. While straightforward to implement, it lacks security as the credentials are not encrypted, making them susceptible to interception.
Digest Authentication
Digest authentication improves upon basic authentication by sending hashed credentials instead of plaintext. This enhances security by reducing the risk of credential interception. However, it still has limitations and may not be suitable for all use cases.
Form-Based Authentication
Form-based authentication involves presenting a web form to the user, prompting them to enter their credentials. Upon submission, the credentials are sent to the server for validation. This method is widely used in web applications and offers flexibility in design and implementation.
OAuth Authentication
OAuth (Open Authorization) is an authentication protocol commonly used for enabling third-party applications to access a user’s resources without revealing their credentials. It involves token-based authentication, where access tokens are exchanged between the client application and the authorization server.
C. Challenges Faced in Automating Authentication with Selenium
Automating authentication with Selenium poses several challenges:
Handling pop-up dialogs: Some authentication mechanisms, such as basic authentication, may prompt users with browser pop-up dialogs, which can be challenging to handle programmatically.
Capturing and managing session cookies: Selenium needs to capture and manage session cookies to maintain authenticated sessions across multiple web pages or tests.
Handling dynamic elements: Web forms and authentication mechanisms may include dynamic elements that change over time or based on user interactions, requiring adaptive automation strategies.
Dealing with CAPTCHA challenges: Websites may employ CAPTCHA challenges to prevent automated access, requiring human intervention or specialized automation techniques to bypass.
Ensuring security: When automating authentication, it’s crucial to ensure that sensitive information, such as credentials and tokens, is handled securely to prevent exposure or unauthorized access.
Addressing these challenges requires careful planning, implementation of robust automation scripts, and adherence to security best practices to ensure the integrity and reliability of automated authentication processes in Selenium tests.
Strategies for Handling Authentication in Selenium
Using WebDriver’s Built-in Features:
WebDriver provides built-in methods to interact with web elements, submit forms, and manage cookies, which can be leveraged to handle various authentication mechanisms.
Pros: Simple and straightforward approach, suitable for many authentication scenarios.
Cons: Limited support for advanced authentication mechanisms like OAuth, may require additional customization for complex scenarios.
Handling Basic Authentication Pop-ups:
Basic authentication pop-ups can be handled using WebDriver’s Alert interface to switch to the alert and provide credentials programmatically.
Pros: Relatively easy to implement for basic authentication scenarios.
Cons: Vulnerable to security risks as credentials are sent in plaintext, may not work for all types of authentication pop-ups.
Handling Form-Based Authentication:
Form-based authentication involves locating and interacting with HTML form elements to submit credentials.
WebDriver’s sendKeys() method can be used to populate username and password fields, followed by submitting the form.
Pros: Flexible approach suitable for a wide range of web applications using form-based authentication.
Cons: Requires detailed knowledge of HTML structure and form elements, may be less effective for dynamically generated forms.
Handling OAuth Authentication:
OAuth authentication involves obtaining and exchanging access tokens between the client application and the authorization server.
Selenium can automate the OAuth flow by interacting with web elements to authorize the application and retrieve access tokens.
Pros: Enables automation of OAuth authentication for third-party integrations.
Cons: Complex to implement due to the multi-step authentication flow and token exchange process, may require advanced scripting and handling of redirects.
Implementing Custom Authentication Handling Solutions:
For complex authentication scenarios not covered by built-in WebDriver features, custom solutions can be developed using programming languages like Java or Python.
Custom solutions may involve intercepting HTTP requests, handling cookies, and managing authentication tokens programmatically.
Pros: Provides flexibility to handle diverse authentication mechanisms and complex scenarios.
Cons: Requires advanced programming skills, increases development effort and maintenance overhead.
Pros and Cons of Each Strategy:
Using WebDriver’s built-in features: Pros – simplicity; Cons – limited support for advanced scenarios.
Handling basic authentication pop-ups: Pros – ease of implementation for basic scenarios; Cons – security risks, limited applicability.
Handling form-based authentication: Pros – flexibility, wide applicability; Cons – requires HTML knowledge, may be less effective for dynamic forms.
Handling OAuth authentication: Pros – automation of third-party integrations; Cons – complexity, requires advanced scripting.
Implementing custom solutions: Pros – flexibility, ability to handle complex scenarios; Cons – requires advanced programming skills, increased development effort.
Choosing the appropriate strategy depends on the specific authentication requirements of the application under test, considering factors such as security, complexity, and maintainability.
Understanding Authorization
Definition and Concept
Authorization is the process of determining what actions a user or system is permitted to perform within a software application or on a specific resource. Once a user has been authenticated, authorization mechanisms enforce access control policies to ensure that only authorized users can access certain functionalities, data, or features. Authorization is typically based on predefined rules, roles, or attributes associated with users or user groups.
Role of Authorization in Web Applications
In web applications, authorization plays a critical role in ensuring data security and protecting sensitive information from unauthorized access. It enables administrators to define and enforce access control policies based on user roles, permissions, or other attributes. By properly configuring authorization settings, web applications can restrict access to confidential data, prevent unauthorized actions, and maintain compliance with regulatory requirements.
Common Authorization Mechanisms
Role-Based Access Control (RBAC):
RBAC is a widely used authorization model that assigns permissions to users based on their roles within an organization. Each role is associated with specific permissions or access rights, allowing administrators to grant or revoke access to resources based on job responsibilities or organizational hierarchy.
Attribute-Based Access Control (ABAC):
ABAC is an authorization model that evaluates access decisions based on attributes associated with users, resources, and environmental conditions. It considers factors such as user attributes (e.g., department, job title), resource attributes (e.g., sensitivity level, ownership), and contextual attributes (e.g., time of access, location).
Role-Based Access Control (RBAC):
RBAC is mentioned twice; it’s probably a mistake. Let’s replace it with another common authorization mechanism:
Role-Based Access Control (RBAC):
In RBAC, access control decisions are based on the roles assigned to users. Each role is associated with a set of permissions, and users are assigned one or more roles based on their job responsibilities or privileges. RBAC simplifies access management by grouping users into roles and defining permissions at the role level.
D. Challenges Faced in Automating Authorization with Selenium
Automating authorization with Selenium presents several challenges:
Identifying and interacting with authorized elements: Selenium needs to locate and interact with web elements that are accessible only to authorized users, which may require dynamically adjusting test scripts based on user roles or permissions.
Handling dynamic content: Web applications often display content dynamically based on user roles or permissions, requiring Selenium scripts to adapt to changes in the UI.
Managing session state: Selenium needs to maintain authenticated sessions across multiple pages or tests to simulate authorized user interactions accurately.
Ensuring data security: Automated tests should not inadvertently access or modify sensitive data that unauthorized users should not have access to, requiring careful test design and validation.
Addressing these challenges requires careful planning, robust test design, and implementation of authorization-aware automation strategies to ensure that Selenium tests accurately reflect the access control policies enforced by the web application.
Strategies for Handling Authorization in Selenium
Accessing and Validating User Permissions:
Selenium can simulate user interactions to access specific functionalities or resources within a web application and then validate whether the expected elements or actions are visible or accessible.
Pros: Direct validation of user permissions, accurate representation of authorized actions.
Cons: Requires detailed knowledge of application functionality and UI elements, may be time-consuming to implement for complex authorization rules.
Verifying Access Control Lists (ACLs):
Selenium can navigate to pages or resources protected by access control lists (ACLs) and verify whether access is granted or denied based on the user’s permissions.
Pros: Ensures compliance with access control policies, validates access to specific resources.
Cons: May require extensive test coverage to validate all ACLs, potential for test maintenance overhead as ACLs change.
Checking Role-Based Permissions:
Selenium can simulate user login with different roles and verify whether the expected functionalities or resources are accessible based on the user’s role.
Pros: Validates role-based access control policies, ensures users are granted appropriate permissions.
Cons: Requires multiple test scenarios for each role, potential for redundancy in test cases.
Handling Authorization Error Messages:
Selenium can verify the presence and content of authorization error messages displayed when users attempt to access unauthorized functionalities or resources.
Pros: Provides feedback on unauthorized access attempts, ensures error handling mechanisms are functioning correctly.
Cons: Limited to verifying error message presence, may not cover all aspects of authorization checks.
Implementing Custom Authorization Handling Solutions:
For complex authorization scenarios not covered by built-in Selenium features, custom solutions can be developed using programming languages like Java or Python.
Custom solutions may involve intercepting HTTP requests, parsing responses, and implementing authorization logic programmatically.
Pros: Provides flexibility to handle diverse authorization mechanisms, addresses complex scenarios.
Cons: Requires advanced programming skills, increases development effort and maintenance overhead.
Pros and Cons of Each Strategy:
Accessing and validating user permissions: Pros – direct validation, accurate representation; Cons – requires detailed knowledge, time-consuming.
Verifying Access Control Lists (ACLs): Pros – ensures compliance, validates access; Cons – extensive test coverage, maintenance overhead.
Checking role-based permissions: Pros – validates access control policies, ensures appropriate permissions; Cons – multiple test scenarios, potential redundancy.
Handling authorization error messages: Pros – provides feedback, ensures error handling; Cons – limited verification scope.
Implementing custom solutions: Pros – flexibility, addresses complex scenarios; Cons – requires advanced skills, increases development effort.
Choosing the appropriate strategy depends on factors such as the complexity of the authorization rules, the level of granularity required in testing, and the available resources for test development and maintenance.
Best Practices for Handling Authentication and Authorization in Selenium
Keep Credentials Secure:
Store credentials securely, such as using encrypted configuration files or secure vaults.
Avoid hardcoding credentials directly into automation scripts.
Implement secure authentication mechanisms, such as OAuth, whenever possible.
Maintain Flexibility in Handling Authentication Mechanisms:
Design automation scripts to handle various authentication methods, including basic authentication, form-based authentication, and OAuth.
Use parameterization to easily switch between different authentication configurations during testing.
Implement Robust Error Handling:
Anticipate and handle authentication and authorization errors gracefully in automation scripts.
Include error logging and reporting mechanisms to capture and troubleshoot authentication failures effectively.
Regularly Update Automation Scripts for Security Changes:
Stay informed about security updates and changes to authentication and authorization mechanisms in the application under test.
Update automation scripts accordingly to adapt to security changes and maintain test reliability.
Collaborate with Development and Security Teams:
Work closely with development and security teams to understand authentication and authorization requirements.
Seek guidance on best practices for securely handling authentication and authorization in Selenium automation.
Document Authentication and Authorization Processes:
Document authentication mechanisms, including configurations and procedures for obtaining access tokens or credentials.
Document authorization policies, including roles, permissions, and access control lists (ACLs) used in the application.
Case Studies and Examples
Demonstrating Authentication Handling with Sample Websites:
Create automation scripts to demonstrate handling authentication scenarios on sample websites with various authentication mechanisms.
Provide step-by-step examples of how to authenticate users using Selenium WebDriver.
Illustrating Authorization Handling with Test Scenarios:
Develop test scenarios to illustrate authorization handling in Selenium, including role-based access control (RBAC) and attribute-based access control (ABAC).
Showcase how Selenium scripts can validate user permissions and access control policies.
Real-world Challenges and Solutions:
Present real-world challenges encountered in handling authentication and authorization in Selenium automation.
Discuss solutions and best practices for addressing common challenges, such as handling dynamic content and managing session state.
Are you seeking Selenium Proxy Job Support? Our service offers expert assistance for seamless integration of Selenium with proxy servers, ensuring efficient automation and robust testing. Gain confidence in your Selenium projects with our dedicated proxy support solutions.
Conclusion
Recap of Key Points:
Authentication and authorization are crucial aspects of Selenium automation for testing web applications securely.
Best practices include keeping credentials secure, maintaining flexibility in handling authentication mechanisms, implementing robust error handling, regularly updating automation scripts, collaborating with development and security teams, and documenting processes.
Final Thoughts on the Importance of Authentication and Authorization in Selenium Automation:
Proper authentication and authorization handling are essential for ensuring the accuracy and reliability of Selenium tests.
By following best practices and leveraging appropriate strategies, testers can effectively simulate real-world user interactions in authenticated environments.
Encouragement for Further Exploration and Learning:
Encourage continued exploration and learning in the field of authentication and authorization in Selenium automation.
Highlight resources and communities where testers can find additional information, share experiences, and collaborate with peers to enhance their skills.