I. Introduction
Importance of Code Maintainability:
In any software development project, code maintainability is crucial for the long-term success and sustainability of the application. As projects evolve, developers often need to add new features, fix bugs, optimize performance, or adapt to changing requirements. Without well-maintained code, these tasks can become increasingly challenging, time-consuming, and error-prone.
Code maintainability encompasses various aspects, including readability, modularity, and ease of debugging. Readable code is easier for developers to understand, modify, and collaborate on. Modular code allows for better organization and separation of concerns, making it simpler to locate and update specific functionality. Additionally, code that is easy to debug can significantly reduce the time and effort required to identify and fix issues.
Introduction to Proxy Objects:
Proxy objects offer a solution to improve code maintainability by providing a flexible and non-intrusive way to add additional behavior or functionality to existing objects or methods. In Java, proxy objects act as intermediaries between a client and the target object, allowing developers to intercept method invocations and execute custom logic before or after the method call.
By using proxy objects, developers can enhance code maintainability in several ways. For instance, they can add cross-cutting concerns such as logging, caching, security checks, or performance monitoring without modifying the original codebase. This promotes better separation of concerns and allows for cleaner, more modular code.
Overall, proxy objects offer a powerful mechanism for improving code maintainability in Java projects by enabling developers to extend or modify the behavior of existing code without directly altering its implementation. In the following sections, we’ll delve deeper into how proxy objects work, their benefits, implementation techniques, and real-world examples of their usage.
II.Understanding Proxy Objects:
Explanation of what proxy objects are and how they work:
Proxy objects are intermediary objects that control access to another object, called the target object. When a client interacts with a proxy object, the proxy intercepts the request and can perform additional actions before or after forwarding the request to the target object. This interception allows for various functionalities like logging, security checks, caching, etc., to be added transparently without modifying the target object’s code.
Types of proxy objects:
- Dynamic Proxies:
Created at runtime, dynamic proxies are generated dynamically based on interfaces and are useful when you need to proxy multiple interfaces or when the interfaces are unknown until runtime.
- Static Proxies:
These are created explicitly by the developer at compile time. They require implementing the same interface as the target object and delegate method calls to the target object.
- Virtual Proxies:
These delay the creation of the actual object until it is needed. They can be useful for resource-intensive objects or objects that require initialization.
- Remote Proxies:
Used for accessing objects in a different address space. Remote proxies hide the complexities of network communication and provide a local representation of the remote object.
Why proxy objects are useful for improving code maintainability:
Proxy objects promote code maintainability by allowing developers to add cross-cutting concerns such as logging, security, or performance monitoring without modifying the original codebase. This ensures that the core functionality remains unchanged and isolated from concerns unrelated to its primary purpose. Additionally, proxy objects facilitate code reuse, modularity, and separation of concerns, leading to cleaner, more maintainable codebases.
III.Benefits of Using Proxy Objects:
Improved code readability and organization:
By encapsulating cross-cutting concerns within proxy objects, the core business logic remains focused and uncluttered. This separation enhances code readability and organization by keeping related functionalities grouped together and isolated from each other.
Separation of concerns and modularization:
Proxy objects promote separation of concerns by allowing developers to address cross-cutting concerns independently from the core functionality. This modularization simplifies code maintenance, as changes to one aspect of the system can be made without impacting other parts.
Simplified debugging and error handling:
Proxy objects facilitate simplified debugging and error handling by providing a centralized location to implement common functionalities such as logging and exception handling. This centralized approach reduces code duplication and ensures consistent error handling across the application.
IV. Use Cases for Proxy Objects:
- Logging:
Proxy objects can be used to add logging functionality to methods without modifying the original code. By intercepting method invocations, logging proxies can log relevant information such as method parameters, return values, and execution times, providing valuable insights for debugging and performance optimization.
- Security:
Proxy objects enable developers to implement security checks such as authentication and authorization without modifying the core functionality of the application. Security proxies intercept method calls and enforce access control policies based on user credentials or other security attributes, ensuring that sensitive operations are performed only by authorized users.
- Performance Monitoring:
Proxy objects can be used to track method execution times and performance metrics, allowing developers to identify bottlenecks and optimize critical sections of the code. Performance monitoring proxies measure the duration of method invocations and record relevant statistics, enabling developers to fine-tune the application for optimal performance.
Use Cases for Proxy Objects:
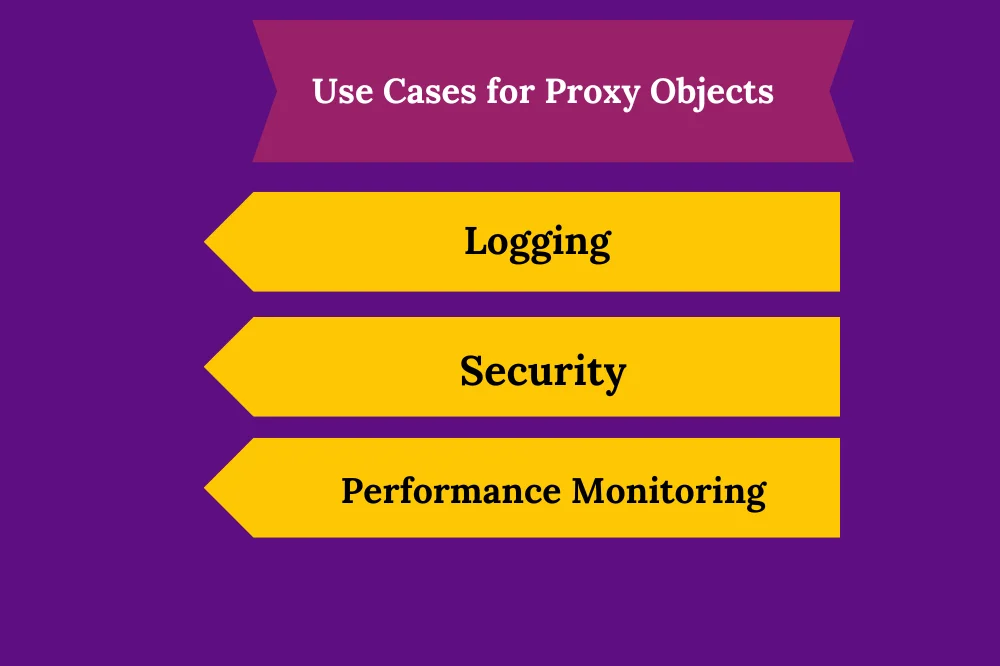
- Logging:
Proxy objects are beneficial for adding logging functionality to applications without cluttering business logic. By intercepting method calls, logging proxies can log relevant information such as method parameters, return values, timestamps, and exceptions. This allows developers to monitor application behavior, diagnose issues, and analyze performance without modifying the original codebase. Logging proxies promote code maintainability by separating logging concerns from business logic, making the codebase cleaner and easier to understand.
- Security:
Proxy objects are useful for implementing security checks in applications without modifying the core functionality. Security proxies intercept method invocations and enforce access control policies based on user credentials, roles, or other security attributes. By centralizing security logic within proxy objects, developers can ensure consistent enforcement of security policies across the application. This promotes code maintainability by isolating security concerns from business logic and facilitating easier maintenance and updates to security policies.
- Performance Monitoring:
Proxy objects can track method execution times and performance metrics to monitor application performance. Performance monitoring proxies measure the duration of method invocations, record relevant statistics, and generate performance reports. By intercepting method calls, performance monitoring proxies provide insights into application performance bottlenecks and help identify areas for optimization. This promotes code maintainability by facilitating proactive performance monitoring and optimization without modifying the original codebase.
V.Implementing Proxy Objects in Java:
Step-by-step guide:
- Define an interface that represents the target object’s functionality.
- Create a proxy class that implements the same interface and delegates method calls to the target object.
- Add custom logic to the proxy class to intercept method invocations and perform additional tasks such as logging, security checks, or performance monitoring.
- Instantiate the proxy object and use it in place of the original target object.
Using Java’s built-in Proxy class for dynamic proxies:
Define an interface or obtain it reflectively.
- Create an invocation handler that implements the InvocationHandler interface and contains the custom logic for intercepting method calls.
- Use the Proxy.newProxyInstance() method to create a dynamic proxy object with the specified interface and invocation handler.
Creating custom proxy classes for more specific use cases:
- Extend the Proxy class or implement the same interface as the target object.
- Implement custom logic in the proxy class to intercept method invocations and perform additional tasks.
- Instantiate the custom proxy object and use it in place of the original target object.
Best Practices and Tips:
Keep proxy logic simple and focused:
- Avoid adding unnecessary complexity to proxy objects.
- Focus on implementing specific functionalities such as logging, security checks, or performance monitoring.
Document proxy functionality and usage:
- Clearly document the purpose and behavior of proxy objects.
- Provide usage examples and guidelines for developers who will be using the proxy objects.
Test proxy objects thoroughly:
- Write comprehensive unit tests to verify the behavior of proxy objects.
- Ensure that proxy objects behave as expected and do not introduce regressions or performance issues.
- Java proxy job support offers expert assistance in Java development, troubleshooting, and project guidance. Our team ensures seamless integration, optimal performance, and efficient problem-solving to bolster your Java projects. Trust us for reliable support tailored to your specific needs.
VI.Conclusion:
In conclusion, proxy objects offer a powerful solution for enhancing code maintainability in Java applications. By encapsulating cross-cutting concerns such as logging, security, and performance monitoring, proxy objects enable developers to modify or extend the behavior of existing code without altering its core implementation. This promotes cleaner, more modular codebases, simplifies debugging and error handling, and facilitates easier collaboration among team members. With proxy objects, developers can achieve better separation of concerns, improve code readability and organization, and streamline maintenance efforts over the lifespan of the application. As a versatile tool in the Java developer’s toolkit, proxy objects contribute to the long-term sustainability and scalability of software projects.