I.Introduction
Brief overview of microservices architecture:
Microservices architecture is an approach to software development where a complex application is broken down into smaller, independent services. Each service, or microservice, is responsible for a specific business function and can be developed, deployed, and scaled independently. This architecture promotes agility, scalability, and flexibility in software development.
Importance of communication between microservices:
In a microservices architecture, communication between services is vital for the system to function properly. Microservices often need to interact with each other to fulfill user requests or perform business processes. Efficient and reliable communication is crucial for maintaining the integrity and functionality of the overall system.
Introduction to Java proxies and their relevance in microservices:
Java proxies are objects that act as intermediaries between a client and a target object. They allow for the interception and manipulation of method invocations on the target object. In the context of microservices, Java proxies can be used to facilitate communication between services by intercepting method calls and adding features such as logging, security, and fault tolerance.
II.Understanding Microservices Architecture
Definition of microservices architecture:
Microservices architecture is an architectural style that structures an application as a collection of loosely coupled services. Each service is self-contained, independently deployable, and communicates with other services through well-defined APIs. This modular approach allows for easier development, deployment, and maintenance of complex systems.
Key characteristics and benefits:
Key characteristics of microservices architecture include service autonomy, decentralized data management, and polyglot persistence. Benefits include improved scalability, flexibility, and resilience. Additionally, microservices enable teams to work independently on different services, promoting faster development cycles and better alignment with business requirements.
Challenges in microservices communication:
Microservices communication introduces challenges such as network latency, service discovery, and distributed data management. Ensuring reliable communication between services while maintaining performance and scalability requires careful design and implementation of communication protocols, error handling mechanisms, and service orchestration strategies.
III.Introduction to Java Proxies
Explanation of what Java proxies are:
Java proxies are objects that dynamically implement interfaces or extend classes at runtime. They intercept method invocations on the target object and allow for additional behavior to be added before, after, or instead of the original method execution. Proxies are commonly used for implementing cross-cutting concerns such as logging, security, and transaction management.
How Java proxies work:
Java proxies work by creating a dynamic proxy class that implements the same interfaces as the target object. Method invocations on the proxy object are intercepted and delegated to an invocation handler, which can perform custom logic before invoking the target method. This mechanism enables transparent interception of method calls without modifying the original source code.
Different types of Java proxies:
There are two main types of Java proxies: dynamic proxies and static proxies. Dynamic proxies are created at runtime using reflection and are suitable for intercepting method calls on interfaces. Static proxies are generated at compile time using code generation tools and require explicit declaration of proxy classes. Both types of proxies have their own advantages and limitations, depending on the use case and requirements.
IV.Role of Java Proxies in Microservices
Proxy pattern in microservices communication:
The proxy pattern is commonly used in microservices architecture to facilitate communication between services. In this pattern, a proxy object acts as an intermediary between the client and the actual service instance. It intercepts requests from the client and forwards them to the appropriate service, adding additional functionality such as logging, authentication, or caching. Proxies help to decouple clients from the implementation details of services, making it easier to modify or replace services without affecting clients.
Handling communication protocols with Java proxies:
Java proxies can be used to handle various communication protocols in microservices architecture, such as HTTP, gRPC, or messaging queues. For example, a proxy can encapsulate the logic for making HTTP requests to remote services, abstracting away the details of network communication from the client code. This allows for more flexibility in choosing and changing communication protocols without impacting clients.
Load balancing and failover mechanisms using proxies:
Java proxies can also be used to implement load balancing and failover mechanisms in microservices architecture. By using proxies as entry points to services, requests can be routed to multiple instances of a service based on load or availability. In case of service failures, proxies can automatically redirect requests to healthy instances, ensuring high availability and reliability of the system.
V.Implementing Java Proxies in Microservices
Example scenario: communication between microservices A and B:
Consider a scenario where microservice A needs to communicate with microservice B to retrieve some data. Instead of directly calling microservice B, microservice A interacts with a proxy object that handles the communication with microservice B on its behalf. This decouples the communication logic from the business logic of microservice A, making the system more modular and maintainable.
Step-by-step implementation using Java proxies:
To implement Java proxies in microservices, you can follow these steps:
- Define an interface that represents the operations supported by the remote service.
- Create a proxy class that implements the interface and delegates requests to the remote service.
- Use a proxy factory or dependency injection to instantiate and configure the proxy object.
- Update client code to use the proxy object instead of directly calling the remote service.
VI.Benefits of Using Java Proxies in Microservices
- Improved scalability and performance:
Java proxies can improve scalability and performance by offloading cross-cutting concerns such as logging, authentication, or caching from service implementations. This allows services to focus on their core functionality, while proxies handle common tasks efficiently.
- Simplified communication between microservices:
Using proxies to encapsulate communication logic between microservices simplifies the development and maintenance of the system. Proxies abstract away the complexities of network communication, making it easier to manage and evolve the communication infrastructure as the system grows.
- Enhanced fault tolerance and resilience:
Java proxies can enhance fault tolerance and resilience by providing mechanisms for load balancing, failover, and circuit breaking. Proxies can automatically reroute requests to healthy instances in case of service failures, improving the overall reliability of the system.
Design considerations when using Java proxies:
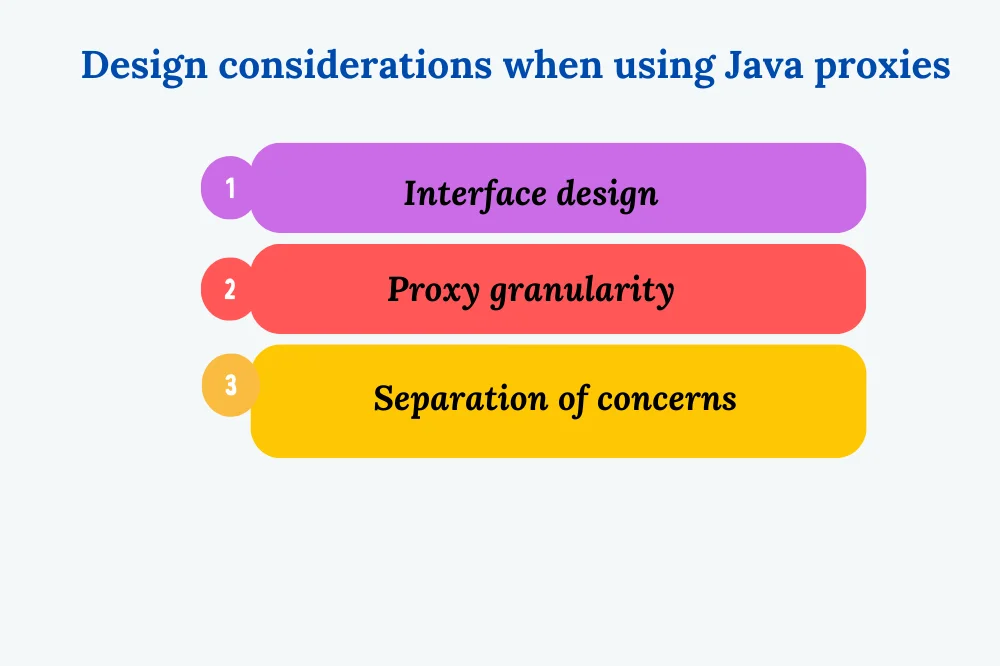
- Interface design: Design clear and concise interfaces for your services to define the contract between the client and the service. This makes it easier to create proxy objects that implement the same interface and ensures compatibility between clients and services.
- Proxy granularity: Consider the granularity of your proxy objects. Depending on the complexity of your system, you may choose to have proxies for individual services, groups of services, or even for cross-cutting concerns such as authentication or logging.
- Separation of concerns: Keep the concerns of your proxies separate from the core business logic of your services. Proxies should primarily handle communication-related tasks such as routing requests, error handling, or adding additional functionality, while the service implementation focuses on its specific functionality.
Monitoring and managing proxy-based communication:
- Logging and tracing: Implement logging and tracing mechanisms in your proxies to monitor communication between services. This allows you to track requests and responses, identify performance bottlenecks, and debug issues more effectively.
- Metrics and monitoring tools: Use metrics and monitoring tools to track key performance indicators (KPIs) such as latency, throughput, and error rates for proxy-based communication. This helps you identify trends, detect anomalies, and optimize system performance.
- Health checks and circuit breaking: Implement health checks and circuit breaking mechanisms in your proxies to monitor the availability and health of remote services. This allows you to gracefully handle failures and prevent cascading failures in your system.
Integration with other microservices tools and frameworks:
- Service discovery: Integrate your proxies with service discovery mechanisms such as Consul, Eureka, or Kubernetes service discovery to dynamically locate and route requests to available instances of remote services.
- API gateways: Consider using API gateways such as Zuul or Kong to centralize and manage communication between clients and services. API gateways can provide additional features such as routing, authentication, rate limiting, and API versioning.
- Message brokers: If your microservices architecture relies on asynchronous communication, integrate your proxies with message brokers such as Kafka, RabbitMQ, or Amazon SQS to decouple producers and consumers of messages and ensure reliable message delivery.
- Java Proxy Job Support offers assistance in developing, maintaining, and troubleshooting proxy-related functionalities in Java applications. Services include proxy server setup, configuration, and optimization for seamless communication between clients and servers.
VII.Conclusion:
In conclusion, Java proxies play a pivotal role in microservices architecture by enabling efficient communication, enhancing scalability, and ensuring resilience. By abstracting away communication complexities and facilitating cross-cutting concerns, proxies simplify system development and maintenance. They allow for seamless integration of services, promote loose coupling, and enable dynamic routing and load balancing. With Java proxies, developers can build robust and flexible microservices architectures that meet the demands of modern applications. In essence, Java proxies serve as a fundamental tool for realizing the benefits of microservices, empowering developers to create agile, scalable, and resilient systems.